Master Python programming
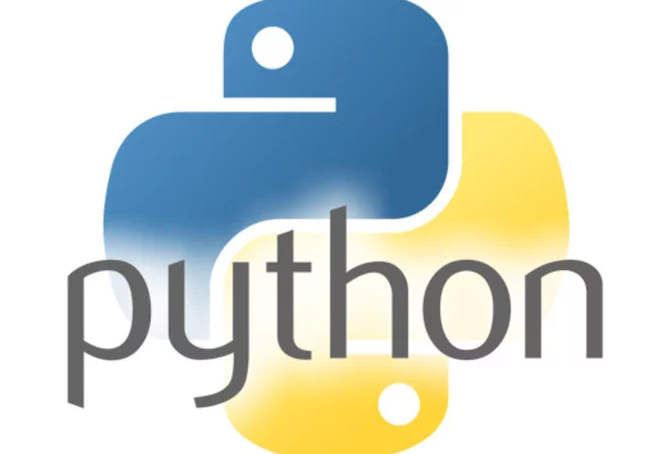
Organizare curs Programare Python
Cursul Master Python Programming: The Complete Python Bootcamp poate fi organizat Online (curs video in Engleza, 100% Hands-On), Corporate customizat sau grupa colectiva. Contacteaza-ne pentru detalii.
Curriculum
Este in limba engleza si contine tutoriale video, demonstratii live, e-book complet, slide-uri, quiz-uri si foarte multe exercitii practice. Accesul la curs se face pe platforma de e-learning Udemy. Click pentru Demo.
obiectiv
La acest curs de programare Python 100% Hands-On vei invata toate conceptele cheie din Python 3, iar la sfasitul cursului vei fi in topul programatorilor Python. Vei putea de asemenea alege specializari de top precum AI, Data Science, Network Automation sau Web Development.
Diploma/Certificare
Dupa promovarea examenelor online vei primi un certificat de absolvire in Limba Engleza care detaliaza cele invatate si contine un calificativ.
Curriculum Curs Programare Python
- Section Introduction
- Installing Python 3 on Windows
- Installing Python 3 on Linux and Mac
- Installing PyCharm IDE on Windows
- Installing PyCharm IDE on Linux and Mac
- Running Python Code using the Python Interpreter
- Running Python Scripts using PyCharm
- Running Python Scripts using the Command Line
Intro to Python
- Compilated Languages vs. Interpretaded Languages
- Setting the Programming Environment (Python, IDLE, PyCharm, VSCode etc)
- Running Python Scripts
- Commnents
- Variables and Constants
- Statically vs. Dynamically Typed Languages
- Python Data Types: numbers (int, float, complex), booleans, strings and data structures
- Working with Numbers
- Booleans
Operators:
- Arithmetic (+, -, *, /, //, **)
- Asignment (=, +=, -=, /=, //=, *=, **=)
- Boolean (==, !=, >, >=, <, <=)
- Identity (is, is not)
- Logical: and, or, not
- Membership: in, not in
- Casting
- Writing Idiomatic Python Code
- Intro to Strings
- Get User Input
- Type Casting
- String Indexing and Operations
- String Slicing
- String Formatting: f-strings, format(), print()
- String Methods
- If Elif and Else Statements
- For Loops
- Ranges In-depth
- For and Continue Statement
- For and Break Statement
- While Loops
- While and continue Statement
- While and break Statement
- Intro to Lists
- List Operations
- List Methods: Append, Extend, Insert, Copy, Clear, Pop, Index, Count, Sort, Max, Min and Sum
- String to List and List to String: Split and Join Methods
- List Comprehension
- Intro to Tuples
- Tuple Operations
- Tuple Methods
- Tuples vs. Lists
- Intro to Sets
- Set Methods Part 1: Add, Clear, Copy, Remove, Discard, Pop
- Set Methods Part 2: Difference, Symmetric Difference, Union, Intersection
- Fronzensets
- Intro to Dictionaries
- Working with Dictionaries
- Dictionary Operations and Methods
- Dictionary Comprehension
- Intro to Functions
- The return Keyword
- Functions Arguments: Positional, Default, Keyword, *args and **kwargs
- Scopes and Namespaces
- One More Detail about Scope and Namespace
- Lambda Expressions
- The Special Constant None
- Opening and Reading Files
- More about Path
- Reading Files: Tell, Seek and Cursors
- The With Keyword
- Reading Files into a List
- Writing to Text Files
- Assignment: File Processing
- Assignment Answer: File Processing
- Intro to Object Oriented Programming
- Defining Classes and Objects
- The __init__ Method
- The __del__ Method
- Instance Attributes and Class Attributes
- Magic Methods
- Intro to Exceptions
- Exceptions Handling: Try…Except…Else…Finally
- Code Debugging
- Intro to Python Modules
- Importing Modules
- Manage External Modules with Pip
- Install External Modules in PyCharm in Venv
- Custom Modules. __name__ and “__main___”
- System-specific Parameters and Functions: The Sys Module
- Script’s Arguments: sys.argv
- Operating System Interfaces: The Os Module
- Project: File Renaming Automation Using Sys and Os Modules
- High-level File Operations: The Shutil Module
- Running System Commands: The Os Module
- Running System Commands: The Subprocess Module
- The Random Module
- Decimal Arithmetic and Decimal Module: Solving the Float Problem
- The Decimal Module: Contexts and Methods
- Intro to CSV
- Reading CSV Files
- Writing CSV Files
- Using Custom Delimiters
- Using CSV Dialects
- Setup the Environment. Installing OpenPyXL
- Excel Basics
- Reading Excel Files
- Reading Data in a Cell Range
- Writing Excel Files
- Creating New Excel Files
- Using Excel Formulas
- Sheets Operations
- Working with Styles
- Intro to Jupyter Notebooks. Installing Jupyter Notebook
- Jupyter Notebook Usage
- Intro to Pandas. Installing Pandas
- Pandas Series
- Pandas DataFrames I. Working with Columns
- Pandas DataFrames I. Working with Rows
- Pandas DataFrames II. Filtering Data
- Reading and Analyzing CSV Files with Pandas
- Reading Excel Files. Groupby and Other Useful Operations
- Reading and Analyzing HTML Pages with Pandas
- Working with Missing Data
- Intro to Python Visualization Libraries
- Installing Plotly
- Creating Scatter Plots
- Creating Line Charts
- Creating Basic Bar Charts
- Creating Grouped and Stacked Bar Charts
- Creating Pie Charts
- Creating Histograms
- Intro to SQLite
- When to Use SQLite
- Connecting to a SQLite DB and Creating Tables with Python
- Inserting with Python
- Selecting with Python
- Parameterized SQL Statements
- Updating with Python
- Deleting with Python
- Intro to Web Scraping using Requests and BeautifulSoup
- Setup the Environment. Installing Requests and BeautifulSoup
- Diving into Requests HTTP Library
- Diving into BeautifulSoup Library
- Project: Real-World Web Scraping (Requests, BeautifulSoup and OpenPyXL)
- Python Parallel Processing Theory
- Multithreading vs. Multiprocessing
- Multithreading and Multiprocessing: Pros and Cons
- Implementing Multiprocessing in Python
- Implementing Multithreading in Python
- Sharing Data Between Processes using Value
- Sharing Data Between Processes using Array
- Implementing Multiprocessing Locks
- Section Introduction
- Download and Install the Required Software: GNS3, VirtualBox, Linux, Cisco Images
- Where do I get Cisco IOS Images
- Just a few Words about Windows Installation
- How to Install GNS3 VM on Windows
- How to run Cisco IOU images on GNS3 VM on Windows
- How to connect to images running in GNS3 from Windows
- Bytes Objects, Encoding and Decoding
- Serial Communication Basics. Connecting to a Console Port
- Open a Serial Connection to a Cisco Device Console Port
- Configure Cisco Devices using Serial Connections
- Telnet Protocol Basics. Configure and Connect to Cisco Devices
- Connecting to Cisco Networking Devices with Telnet from Python
- Getpass Module
- Network Automation using Telnet. Configure Multiple Cisco Devices
- Intro to Paramiko
- Bonus: Enable SSH on Cisco Devices
- Running a Command on a Cisco Device using Paramiko(SSH)
- Running a Command on Linux using Paramiko(SSH)
- Configure Loopback and OSPF on a Cisco Router using Paramiko(SSH)
- Secure Copying Files to Linux with SCP and Paramiko from Python
- Intro to Netmiko
- Connecting and Running a Command on a Cisco Networking Device
- Netmiko prompt. Enable & Global Config Mode
- Running Multiple Commands on a Cisco Networking Device
- Configure a Cisco Networking Device from a File
- Configure Multiple Cisco Networking Devices from Multiple Files
- Configure Backup using Netmiko
- Improvement of the Configuration Backup Script
- Netmiko and Linux
- Troubleshooting Netmiko
- Assignment: Check Interface Status and Enable it if it’s Disabled
- Assignment Answer Check Interface Status and Enable it if it’s Disabled
Intrebari frecvente (faq)
Daca depui mult efort si pasiune poti deveni un programator foarte bun. Vei pasi intr-o lume cu somaj zero la nivel mondial, vei avea un job universal pe care-l poti practica in orice tara, vei fi independent si vei depinde doar de propriile cunostinte, iar salariul va fi mereu peste medie.
Se preconizeaza ca programarea este jobul viitorului, iar viata noastra va fi din ce in ce mai legata de aplicatii. Python este limbajul recomandat cu care sa incepi. Crezi ca merita ?
Da. Python este al 3-lea cel mai folosit limbaj de programare din lume in 2019, fiind limbajul cu cea mai spectaculoasa crestere. Domenii de top precum Inteligenta Artificiala, Machine Learning, Data Science, sau Network Automation folosesc Python intensiv.
Da. Python este un limbaj perfect pentru incepatori. Este concis si usor de inteles. De fapt universitatile din USA si Europa au inlocuit Java cu Python ca prim limbaj.
Cursul Master Python Programming: The Complete Python Bootcamp se desfasoara in limba engleza pe Udemy – cea mai mare platforma de e-learning din lume.
Pentru a te inscrie la cursul de Programare Python (Master Python Programming: The Complete Python Bootcamp) nu trebuie sa ai cunostinte initiale de programare sau Python. Cursul incepe de la zero. Trebuie sa ai insa cunostinte foarte bune de folosire a calculatorului in general precum si cunostinte medii de limba engleza. Cursul se desfasoara in engleza.
Acest curs este video 100% Hands-on si nu este “doar un alt curs de programare Python”. Este singurul curs de care ai nevoie pentru a deveni un programator de top. Dovada stau cei peste 1000 de cursanti precum si review-urile pozitive primite. In plus poti urmari mai multe sectiuni demo inainte de a te inscrie.
Ce spun cursantii care s-au inscris pana acum
Daca vreti sa invatati Python nu mai cautati alt curs, acesta este cursul pe care trebuie sa-l faceti"